
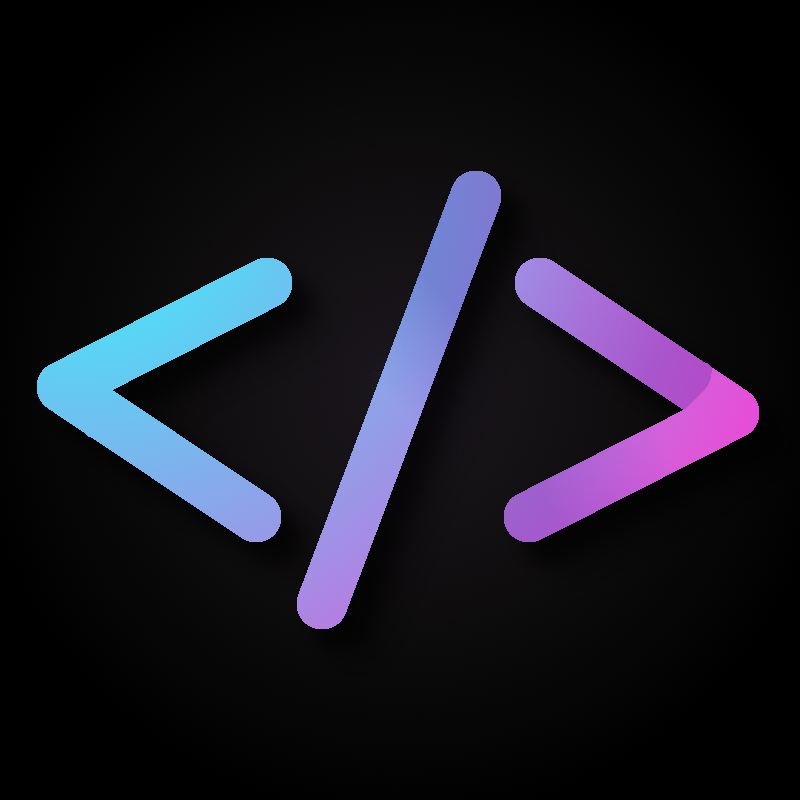
C# is a great language, I don’t know much about game dev but I know unity and godot game engines have good support for c#. You can target Windows/Linux/Mac on all the common architectures. All the build tools are available on the command line if that’s your thing.
Ok, I’m in for $2 /month too, thanks for setting the standard!